
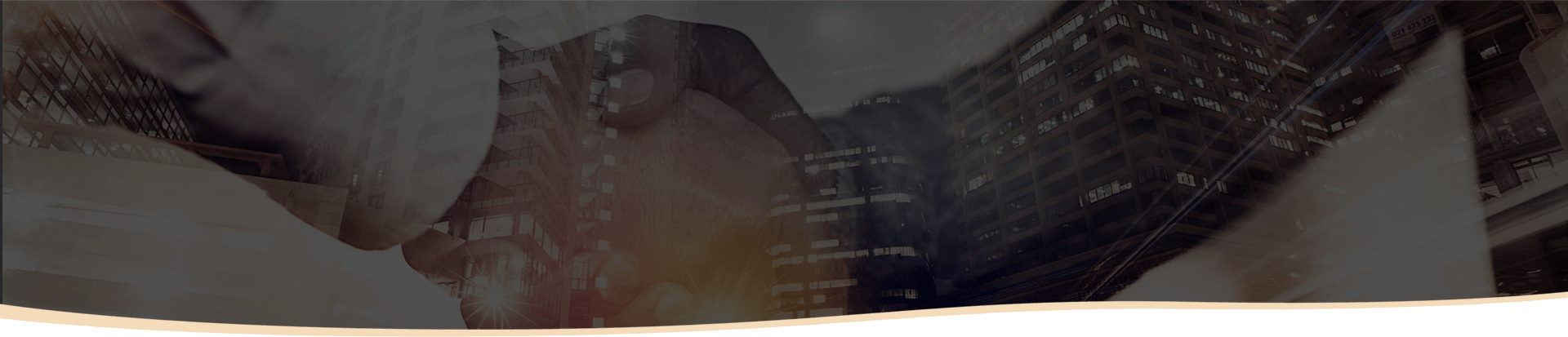
API Guide
Parameters are passed in JSON format
headers block is required for all types of requests
Example:
headers {
Content-Type: application/json
Authorization: Bearer TOKEN (receive after authorization)
}
Available endpoints:
Log In
Example:
Method: POST
EndPoint: {{"https://api1.adataprotect.com/api/user/login"}}
Params:
login - string - your login
password - string - your password
Response:
in case of success:
{
“result” : {
“token” : “TOKEN”
in case of error:
{
“errors” : [
..// Errors (example: 'parameter is missing: validity')
]
or
{
“errors” : “Server Error - {message}”
Tool Signature Request:
Example:
Method: POST
EndPoint: /api/transaction/creates/payments
Params:
payer_id - string - your id in our system (check with the admin)
owner - string - Payer's name (From the card)
card_number - string - Card number (From the card)
cvv - integer - number on the back of the card (From the card)
validity - string - card expiry date. Example: “01/22” (From card)
amount - float/integer - write-off amount
currency - string - currency . Example: "USD", always in upper case.
Response:
in case of success:
{
“result” : {
“transaction” : “transaction number” ,(99681628714189)
“status”: 1|2|3|5,
“redirect_url”: “http://exemple.com/…..” (3DS, option)
*redirect_url - url to redirect the user to pass 3DS.
after passing the 3DS, the user will be redirected to the authentication completion page.
Possible statuses:
STATUS_IN_PROCESS = 1;
STATUS_APPROVED = 2;
STATUS_DENIED = 3;
STATUS_WAITING_ CONFIRMATION = 5;
“status”: “STATUS_IN_PROCESS” - Transaction in progress, check status after a while
“status”: “STATUS_APPROVED” - Transaction completed
“status”: “STATUS_DENIED” - The transaction was NOT completed due to reasons beyond our control
“status”: “STATUS_WAITING_ CONFIRMATION” - waiting for confirmation from the user
in case of error:
{
“errors” : [
..// Errors (example: 'parameter is missing: validity')
]
or
{
“errors” : “Server Error - {message}”
Transaction status request:
Example:
Method: GET
EndPoint: api/transaction/transactions/ {id}/status
Params:
id - string - transaction number (you receive in the response with a successful request to withdraw funds)
Response:
in case of success:
{
“result” : {
“status” : (int) transaction status
Possible statuses:
STATUS_IN_PROCESS = 1;
STATUS_APPROVED = 2;
STATUS_DENIED = 3;
STATUS_REFUND = 4;
STATUS_WAITING_ CONFIRMATION = 5;
in case of error:
{
“errors” : [
..// Errors (example: 'parameter is missing: validity')
]
or
{
“errors” : “Server Error”
Refund:
Example:
Method: POST
EndPoint: /api/transaction/refunds
Params:
payer_id - string - your id in our system (check with the admin)
transaction_id - string - transaction number
Response:
in case of success:
{
“result” : "OK"
}
in case of error:
{
“errors” : “Query params is not valid”
or
{
“errors” : “Query params is not valid”
or
{
“errors” : “Sever Error - {message}”
Transaction info:
Example:
Method: GET
EndPoint: https://api1.adataprotect.com/api/transaction/transactions/{transaction number}/info
Response:
in case of success:
{
“result” : {
"transaction_status": 3,
"transaction_amount": 0.01,
"net_amount": 0.01,
"transaction_date_time": "2021-11-22 08:40:40 UTC",
"is_live_transaction": true,
"card_owner_name": "Test Test",
"card_number": "6690"
in case of error:
{
“errors” : “Transaction is not found”
Transactions list:
Example:
Method: POST
EndPoint: https://api1.adataprotect.com/api/transaction/all/transactions/info
Params:
payer_id - string - your id in our system (check with the admin)
isLiveTransaction - string - optional (flag test transaction or not)
startDate - string - optional (passing date in timestamp format)
endDate - string - optional (passing date in timestamp format)
Response:
in case of success:
{
“result” :
[
"transaction_number": "94621337271640",
"transaction_status": 3,
"transaction_amount": 0.01,
"net_amount": 0.01,
"transaction_date_time": "2021-11-22 08:40:40 UTC",
"is_live_transaction": true,
"card_owner_name": "Test Test",
"card_number": "6690"
}
in case of error:
{
“errors” : “Missing params”